[문제]
src.txt라는 파일을 만들고 아래와 같이 입력하고 저장한다.
Hello C World
Welcome to C World
src.txt 파일을 바이너리 데이터로 읽어서 des에 바이너리 데이터로 쓰는 바이너리 파일 복사 프로그램을 작성하라.
조건1) 파일을 여는 데 실패하면 메시지를 띄울 것
조건2) 파일을 복사하는데 성공하거나 실패하면 그에 따른 메시지를 띄울 것
조건3) memset 함수를 사용하지 말 것
[실행결과]
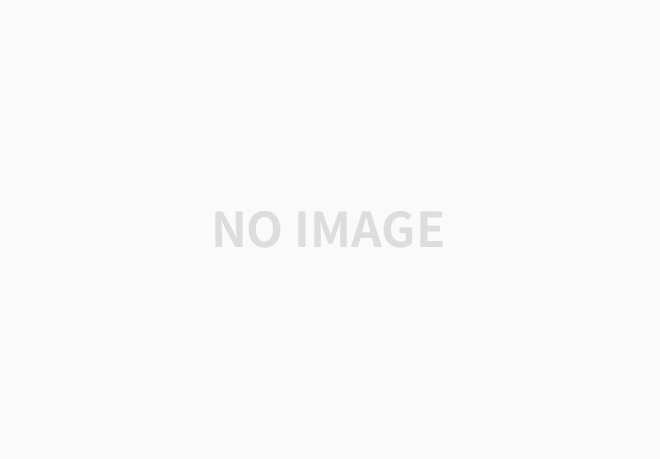
[수정할 코드]
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 30
int main(void)
{
char buffer[BUFFER_SIZE];
int res;
FILE* fp1 = fopen("src.txt", "rb");
FILE* fp2 = fopen("dst.txt", "wb");
if (fp1 == NULL || fp2 == NULL) {
puts("파일오픈 실패");
return -1;
}
while (1)
{
res = fread(buffer, 1, BUFFER_SIZE, fp1);
if (res != BUFFER_SIZE) {
if (feof(fp1) != 0) {
fwrite(buffer, 1, BUFFER_SIZE, fp2);
puts("file copy complete");
}
else {
puts("file copy fail");
}
break;
}
fwrite(buffer, 1, BUFFER_SIZE, fp2);
memset(buffer, 0x0, sizeof(buffer));
}
fclose(fp1);
fclose(fp2);
return 0;
}
[코드]
#include<stdio.h>
int main(void)
{
int readCnt;
char buf[20];
FILE* fp1 = fopen("src.txt", "rb");
FILE* fp2 = fopen("des.txt", "wb");
if (fp1 == NULL || fp2 == NULL) {
puts("파일오픈 실패");
return -1;
}
while (1)
{
readCnt = fread((void*)buf, 1, sizeof(buf), fp1);
if (readCnt < sizeof(buf))
{
if (feof(fp1) != 0)
{
fwrite(buf, 1, readCnt, fp2);
printf("파일 복사 완료");
break;
}
else
printf("파일 복사 실패");
break;
}
fwrite((void*)buf, 1, sizeof(buf), fp2);
}
fclose(fp1);
fclose(fp2);
return 0;
}
<참고>
윤성우의 열혈 C 프로그래밍
'프로그래밍 언어 문제 > C' 카테고리의 다른 글
[C언어] 연습문제5 (0) | 2022.04.20 |
---|---|
[C언어] 연습문제6 (0) | 2022.04.16 |
[C언어] 연습문제8 (0) | 2022.04.13 |
[C언어] 연습문제9 (0) | 2022.04.13 |
[C언어] 연습문제10 (0) | 2022.04.08 |